Contents
Reinforcement learning program features
Reinforcement learning (RL) is a subfield of machine learning where an agent learns to make sequential decisions by interacting with an environment. RL programs have several distinctive features:
- Sequential Decision-Making: In reinforcement learning, an agent makes a series of decisions over time. These decisions affect the agent’s future experiences and rewards, creating a sequential decision-making process.
- Agent-Environment Interaction: The RL agent interacts with an environment. It takes actions in the environment, receives feedback in the form of rewards or penalties, and observes the state of the environment, which can be partial or fully observable.
- Rewards: Rewards are numerical values that the agent receives from the environment as feedback for its actions. The agent’s objective is to maximize the cumulative reward over time.
- Exploration vs. Exploitation: The agent faces a trade-off between exploration (trying new actions to learn more about the environment) and exploitation (choosing actions that are believed to yield high rewards based on current knowledge).
- Markov Decision Process (MDP): RL problems are often modeled as Markov Decision Processes, which have states, actions, transition probabilities, and reward functions. MDPs provide a formal framework for RL tasks.
- Policy: The policy is a strategy or a mapping from states to actions that defines the agent’s behavior. The agent aims to learn an optimal policy that maximizes its expected cumulative reward.
- Value Functions: Value functions, such as the state-value function (V) and action-value function (Q), provide estimates of the expected cumulative reward under a given policy. These functions help the agent make better decisions.
- Exploration Strategies: RL agents use various exploration strategies to decide which actions to take. Common strategies include epsilon-greedy, softmax, and UCB (Upper Confidence Bound).
- Model vs. Model-Free RL: In some RL algorithms, the agent builds a model of the environment (model-based RL) to plan and make decisions. In others, the agent directly learns from experience (model-free RL).
- Algorithms: There are various RL algorithms, including Q-learning, SARSA, Deep Q-Networks (DQN), Policy Gradient methods, and Actor-Critic methods. The choice of algorithm depends on the problem’s characteristics.
- Function Approximation: In many RL applications, especially deep reinforcement learning, function approximation techniques like neural networks are used to estimate value functions or policies.
- Credit Assignment: Credit assignment is the challenge of attributing the outcomes (rewards) of a sequence of actions to the actions themselves. This is a fundamental problem in RL.
- Continuous vs. Discrete Actions and States: RL problems can involve continuous or discrete action and state spaces, and different algorithms are suited to different types of problems.
- Episodic vs. Continuous Tasks: RL tasks can be episodic, where episodes have a fixed length and terminate, or continuous, where the agent interacts with the environment indefinitely.
- Exploration Challenges: Balancing exploration and exploitation can be challenging, especially in high-dimensional or continuous action spaces.
- Sample Efficiency: RL algorithms often require many interactions with the environment to learn effectively, which can be computationally expensive.
- Transfer Learning: RL agents may be able to transfer knowledge learned in one environment to accelerate learning in a related task.
- Safety and Ethics: RL applications in real-world domains must consider safety and ethical considerations, as agents may learn undesirable behaviors.
- Evaluation Metrics: RL performance is typically evaluated using metrics like the cumulative reward, learning curve, and success rate.
Reinforcement learning is a powerful paradigm for solving problems where agents must learn to make decisions in complex and dynamic environments. It finds applications in robotics, game playing, autonomous systems, recommendation systems, and more. However, RL also presents unique challenges, such as exploration, credit assignment, and sample efficiency, which require careful consideration when designing RL programs.
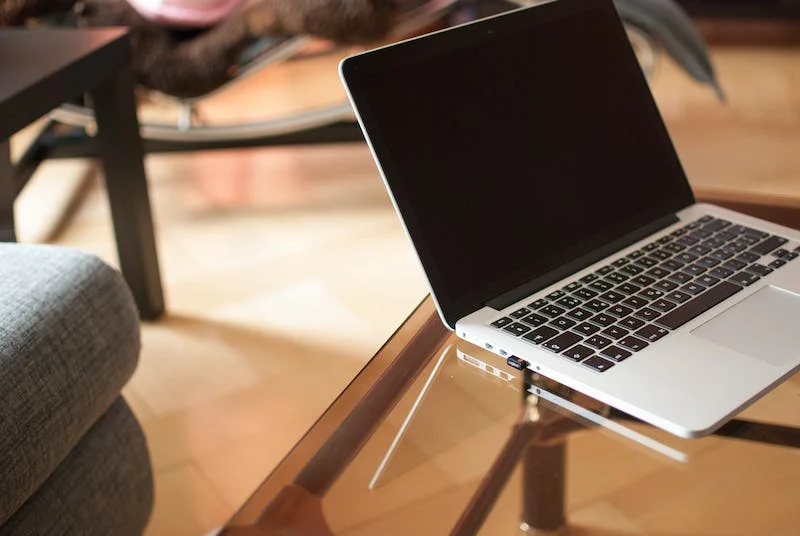
Machine Learning, Reinforcement learning coding examples
Certainly! Here are some reinforcement learning coding examples using Python and the OpenAI Gym library, which is a popular toolkit for developing and comparing reinforcement learning algorithms:
Example 1: Q-Learning for FrozenLake
In this example, we’ll use Q-Learning to solve the FrozenLake environment in OpenAI Gym. FrozenLake is a gridworld environment where the agent must navigate through icy terrain to reach a goal.
pythonCopy codeimport gym
import numpy as np
# Create the FrozenLake environment
env = gym.make('FrozenLake-v1', is_slippery=False) # Set 'is_slippery' to True for a more challenging version
# Initialize the Q-table
Q = np.zeros([env.observation_space.n, env.action_space.n])
# Set hyperparameters
learning_rate = 0.1
discount_factor = 0.99
num_episodes = 1000
# Q-Learning algorithm
for episode in range(num_episodes):
state = env.reset()
done = False
while not done:
# Choose an action using epsilon-greedy policy
if np.random.rand() < 0.1:
action = env.action_space.sample() # Exploration
else:
action = np.argmax(Q[state, :]) # Exploitation
# Take the chosen action and observe the next state and reward
next_state, reward, done, _ = env.step(action)
# Update the Q-table
Q[state, action] = (1 - learning_rate) * Q[state, action] + \
learning_rate * (reward + discount_factor * np.max(Q[next_state, :]))
state = next_state
# Evaluate the trained Q-table
num_episodes_eval = 100
num_successful_episodes = 0
for episode in range(num_episodes_eval):
state = env.reset()
done = False
while not done:
action = np.argmax(Q[state, :])
next_state, reward, done, _ = env.step(action)
state = next_state
if done and reward == 1:
num_successful_episodes += 1
success_rate = num_successful_episodes / num_episodes_eval
print(f"Success Rate: {success_rate * 100:.2f}%")
Example 2: Deep Q-Network (DQN) for CartPole
In this example, we’ll implement a Deep Q-Network (DQN) to solve the CartPole environment, where the agent must balance a pole on a moving cart.
pythonCopy codeimport gym
import numpy as np
import tensorflow as tf
from tensorflow.keras import layers
# Create the CartPole environment
env = gym.make('CartPole-v1')
# Define the DQN model
model = tf.keras.Sequential([
layers.Dense(24, activation='relu', input_shape=(env.observation_space.shape[0],)),
layers.Dense(24, activation='relu'),
layers.Dense(env.action_space.n, activation='linear')
])
# Define optimizer and loss function
optimizer = tf.keras.optimizers.Adam(learning_rate=0.001)
loss_fn = tf.keras.losses.mean_squared_error
# Set hyperparameters
discount_factor = 0.95
epsilon = 1.0
epsilon_decay = 0.995
min_epsilon = 0.01
batch_size = 64
num_episodes = 1000
# DQN algorithm
for episode in range(num_episodes):
state = env.reset()
done = False
while not done:
# Choose an action using epsilon-greedy policy
if np.random.rand() < epsilon:
action = env.action_space.sample() # Exploration
else:
q_values = model.predict(np.expand_dims(state, axis=0))
action = np.argmax(q_values) # Exploitation
# Take the chosen action and observe the next state and reward
next_state, reward, done, _ = env.step(action)
# Update replay buffer and train the DQN model
# (Implementing experience replay and target network is recommended for stability)
state = next_state
# Decay epsilon
epsilon = max(min_epsilon, epsilon * epsilon_decay)
# Evaluate the trained DQN
num_episodes_eval = 100
num_successful_episodes = 0
for episode in range(num_episodes_eval):
state = env.reset()
done = False
while not done:
q_values = model.predict(np.expand_dims(state, axis=0))
action = np.argmax(q_values)
next_state, _, done, _ = env.step(action)
state = next_state
if done:
num_successful_episodes += 1
success_rate = num_successful_episodes / num_episodes_eval
print(f"Success Rate: {success_rate * 100:.2f}%")
These examples demonstrate reinforcement learning using Q-Learning and Deep Q-Network (DQN) for different environments in OpenAI Gym. Keep in mind that RL can be complex, and these are simplified examples. In practice, you may need more advanced techniques like experience replay, target networks, and exploration strategies for more challenging tasks.