Contents
Kotlin development language features
Kotlin is a modern, statically-typed programming language that has gained popularity for its conciseness, safety, and interoperability with Java. It offers a range of language features that make it a compelling choice for Android app development and other application development scenarios. Here are some of the key language features of Kotlin:
- Conciseness: Kotlin is known for its concise syntax, which reduces boilerplate code. For example, you don’t need to declare getters and setters for properties, and semicolons are often optional.
- Null Safety: Kotlin addresses the issue of null references at the language level. You must explicitly specify when a variable can be null using the
?
modifier, and the compiler enforces null safety, reducing the likelihood of NullPointerExceptions. - Type Inference: Kotlin has a strong type system, but it often infers types, which reduces the need for explicit type declarations.
- Extension Functions: Kotlin allows you to add new functions to existing classes without modifying their source code. This is a powerful feature for enhancing the functionality of libraries and classes.
- Smart Casts: Kotlin uses smart casts to automatically cast a variable to the appropriate type when certain conditions are met. This reduces the need for explicit type casting.
- Data Classes: Data classes in Kotlin are used for creating simple classes to hold data. The compiler automatically generates standard methods like
equals()
,hashCode()
, andtoString()
for data classes. - Coroutines: Kotlin provides built-in support for coroutines, which simplifies asynchronous programming. Coroutines allow you to write asynchronous code in a sequential, easy-to-read style.
- Lambda Expressions: Kotlin makes it easy to work with higher-order functions, including lambda expressions. You can pass functions as arguments, return them from other functions, and store them in variables.
- Immutability: You can declare immutable variables using the
val
keyword, and mutable variables using thevar
keyword. This encourages safer programming practices. - Ranges: Kotlin provides built-in support for creating ranges of values. This is particularly useful for iterating over a range of numbers or characters.
- Interoperability with Java: Kotlin is fully interoperable with Java. You can use Java libraries in Kotlin code, and vice versa. This makes it a smooth transition for developers coming from a Java background.
- Infix Functions: Kotlin allows you to define infix functions, which can make your code more readable, especially when working with domain-specific languages.
- Type Aliases: You can create type aliases in Kotlin to provide more descriptive names for complex types, improving code readability.
- Companion Objects: Kotlin has companion objects associated with classes, which are similar to static methods and properties in Java.
- Sealed Classes: Sealed classes are used to represent restricted class hierarchies. They are often used in conjunction with when expressions for exhaustive checks.
- Property Delegation: Kotlin provides a way to delegate property handling to other classes, making it easier to manage properties and their behavior.
These are just some of the notable features of Kotlin that make it a powerful and expressive programming language for a wide range of applications, particularly for Android development.
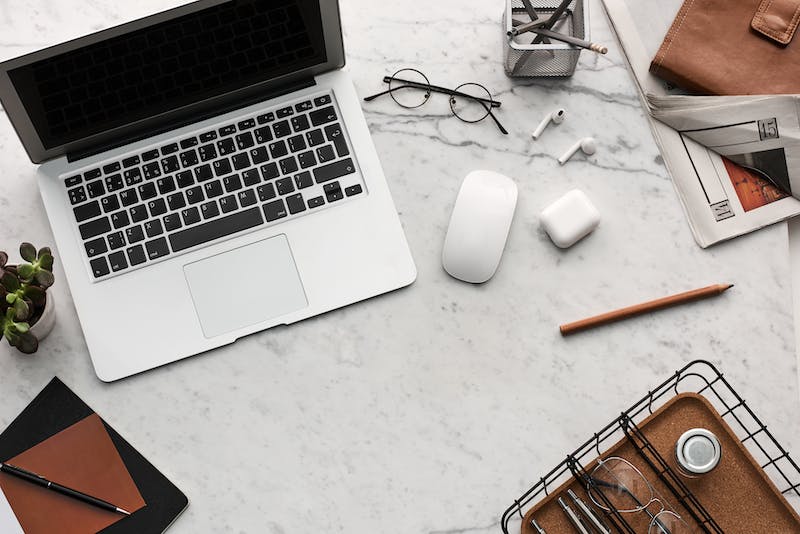
Kotlin development language, sign-up code sample
Creating a mobile sign-up feature in Kotlin involves several components, including UI layout, user input validation, and network communication. Below is a simplified Kotlin code sample for a mobile sign-up screen that demonstrates the basic structure and functionality. This example is for educational purposes and should be adapted and extended for production use.
This code assumes you have a basic Android project set up with a layout XML file for the sign-up screen. Here’s how the Kotlin code might look:
kotlinCopy code// Import necessary Android classes and libraries
import android.app.Activity
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
import android.widget.Toast
class SignUpActivity : Activity() {
// Declare UI elements
private lateinit var usernameEditText: EditText
private lateinit var emailEditText: EditText
private lateinit var passwordEditText: EditText
private lateinit var signUpButton: Button
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_sign_up) // Set the layout XML
// Initialize UI elements
usernameEditText = findViewById(R.id.editTextUsername)
emailEditText = findViewById(R.id.editTextEmail)
passwordEditText = findViewById(R.id.editTextPassword)
signUpButton = findViewById(R.id.buttonSignUp)
// Set a click listener for the sign-up button
signUpButton.setOnClickListener {
val username = usernameEditText.text.toString()
val email = emailEditText.text.toString()
val password = passwordEditText.text.toString()
if (validateInput(username, email, password)) {
// If input is valid, you can proceed with the sign-up process here
// For example, make a network request to create a new user account
// and handle success or error responses accordingly
// You may use libraries like Retrofit for network requests
// Example: performSignUp(username, email, password)
}
}
}
private fun validateInput(username: String, email: String, password: String): Boolean {
// Perform basic input validation here
if (username.isEmpty() || email.isEmpty() || password.isEmpty()) {
showToast("Please fill in all fields")
return false
}
// Add more validation checks as needed (e.g., email format, password strength)
return true
}
private fun showToast(message: String) {
Toast.makeText(this, message, Toast.LENGTH_SHORT).show()
}
// You can define a function for making the network request to sign up the user
// private fun performSignUp(username: String, email: String, password: String) {
// // Implement the sign-up logic here, e.g., using Retrofit to communicate with an API
// }
}
In this code:
- We initialize UI elements such as
EditText
fields for username, email, and password, and aButton
for sign-up. - In the
onCreate
method, we set a click listener for the sign-up button, where we retrieve user input and validate it using thevalidateInput
function. You can add more complex validation rules as needed. - If the input is valid, you can proceed with the sign-up process, such as making a network request to create a new user account. The
performSignUp
function is a placeholder for this action, which you can implement using a networking library like Retrofit. - The
showToast
function is used to display messages to the user for feedback.
Please note that this is a simplified example, and a complete production-ready sign-up process would include additional features such as password encryption, error handling, and user feedback during the registration process.
Kotlin development language, search code sample
Creating a mobile search feature in Kotlin for Android typically involves a combination of UI components, search functionality, and adapter-based list views. Below is a simplified Kotlin code sample that demonstrates a basic search feature within an Android app.
This example assumes you have a basic Android project set up with a list of items that you want to search through. You can adapt and extend this code for your specific use case.
Here’s a simplified code sample:
kotlinCopy code// Import necessary Android classes and libraries
import android.app.Activity
import android.os.Bundle
import android.widget.ArrayAdapter
import android.widget.EditText
import android.widget.ListView
import android.widget.Toast
import java.util.*
class SearchActivity : Activity() {
// Declare UI elements
private lateinit var searchEditText: EditText
private lateinit var listView: ListView
// Sample data for demonstration
private val data = listOf("Apple", "Banana", "Cherry", "Date", "Grapes", "Lemon", "Mango", "Orange", "Pineapple")
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_search) // Set the layout XML
// Initialize UI elements
searchEditText = findViewById(R.id.editTextSearch)
listView = findViewById(R.id.listView)
// Initialize and populate the list view with data
val adapter = ArrayAdapter(this, android.R.layout.simple_list_item_1, data)
listView.adapter = adapter
// Set a text change listener for the search EditText
searchEditText.addTextChangedListener(object : TextWatcher {
override fun beforeTextChanged(s: CharSequence?, start: Int, count: Int, after: Int) {
// Not used in this example
}
override fun onTextChanged(s: CharSequence?, start: Int, before: Int, count: Int) {
// Filter the list view data based on the search query
val query = s.toString().toLowerCase(Locale.getDefault())
adapter.filter.filter(query)
}
override fun afterTextChanged(s: Editable?) {
// Not used in this example
}
})
// Set a click listener for list view items
listView.setOnItemClickListener { parent, view, position, id ->
val selectedItem = parent.getItemAtPosition(position).toString()
showToast("Selected: $selectedItem")
}
}
private fun showToast(message: String) {
Toast.makeText(this, message, Toast.LENGTH_SHORT).show()
}
}
In this code:
- We initialize UI elements, including an
EditText
for search input and aListView
to display search results. - Sample data is provided for demonstration purposes. In a real application, you would replace this with your own data source.
- We set up an
ArrayAdapter
to populate theListView
with the initial data. - We add a text change listener to the
searchEditText
. As the user types, it filters the data in the list view based on the search query. - When the user clicks on an item in the list view, a message is displayed to indicate the selected item.