Contents
React Native development language features
React Native is a popular open-source framework for building mobile applications using JavaScript and React. It allows developers to build cross-platform mobile applications with a single codebase.
- JavaScript: React Native primarily uses JavaScript as its programming language. Developers can write their application logic in JavaScript, which is a widely used and well-supported language.
- React: React is a JavaScript library for building user interfaces, and React Native extends the concepts of React to mobile app development. You use components to build the user interface, and React’s virtual DOM ensures efficient updates.
- ES6 and Beyond: React Native encourages the use of modern JavaScript features, including ES6 and ES7 syntax, which provides enhanced readability and maintainability of code.
- Components: React Native applications are built using reusable UI components. You can create custom components or use built-in ones like
View
,Text
, andImage
. - Props: Components can receive data through props (short for properties). This allows you to pass information from parent components to child components.
- State: State is used to manage data that can change over time in your application. Components can have local state, and you can use the
useState
hook to manage it. - Styles: React Native uses a stylesheet system similar to CSS, where you define the styles for your components. It uses Flexbox for layout, making it easy to create responsive UIs.
- Navigation: Navigation is an important aspect of mobile app development. React Navigation is a popular library for handling navigation in React Native applications.
- Async and Await: React Native applications often interact with remote servers or databases, so asynchronous programming with
async
andawait
is frequently used. - Redux or Mobx (optional): For managing the application’s state, you can use state management libraries like Redux or Mobx. These libraries help you centralize and manage application state in a predictable manner.
- API Integration: You’ll often make network requests to fetch data from APIs. Libraries like Axios or the built-in
fetch
API are commonly used for this purpose. - Native Modules: React Native allows you to write native code (Java, Swift, Objective-C) to interact with native device functionality that might not be accessible through JavaScript alone. This can be done through the creation of native modules.
- Debugging Tools: React Native provides debugging tools, such as React DevTools and the in-browser console, as well as remote debugging using tools like React Native Debugger.
- Hot Reloading: React Native supports hot reloading, which allows you to see the results of your code changes immediately in the running app, making the development process faster and more efficient.
- Third-party Libraries: React Native has a vast ecosystem of third-party libraries and packages available through npm that can extend the functionality of your app.
- Cross-Platform Development: React Native allows you to write code once and deploy it on both iOS and Android, with platform-specific code when necessary.
These language features and concepts are essential for developing mobile applications using React Native. Learning and mastering these concepts will enable you to create efficient and responsive cross-platform mobile apps.
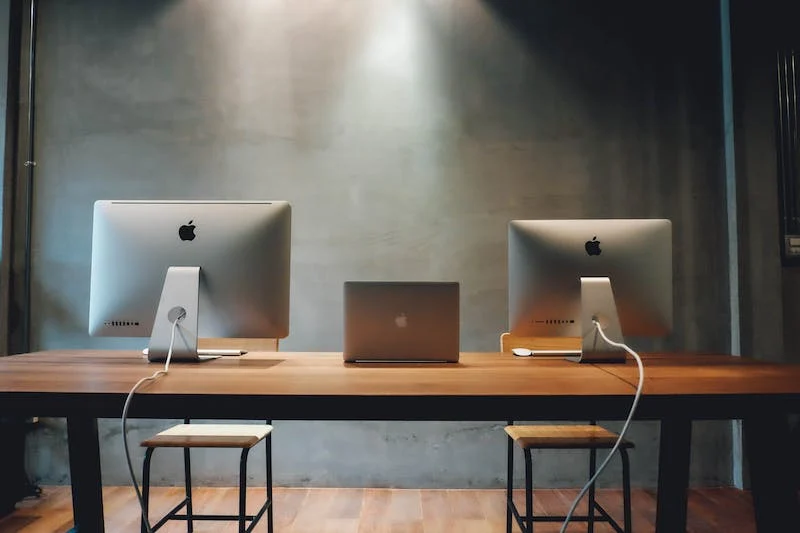
React Native, sign-up code sample
Creating a mobile sign-up form in React Native involves using various components, handling user input, and potentially making API requests to register a user. Below is a simple code sample for a mobile sign-up form using React Native and React Navigation. This code assumes you have already set up a React Native project and have React Navigation installed. You may need to adjust it to your specific project structure and styling preferences.
jsxCopy codeimport React, { useState } from 'react';
import { View, Text, TextInput, Button, StyleSheet } from 'react-native';
const SignUpScreen = ({ navigation }) => {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const handleSignUp = () => {
// You can add validation, API calls, and user registration logic here
// For simplicity, we're just logging the input values
console.log('Email:', email);
console.log('Password:', password);
// After successful registration, navigate to another screen
navigation.navigate('Home');
};
return (
<View style={styles.container}>
<Text style={styles.label}>Email</Text>
<TextInput
style={styles.input}
onChangeText={text => setEmail(text)}
value={email}
placeholder="Enter your email"
/>
<Text style={styles.label}>Password</Text>
<TextInput
style={styles.input}
onChangeText={text => setPassword(text)}
value={password}
secureTextEntry={true}
placeholder="Enter your password"
/>
<Button title="Sign Up" onPress={handleSignUp} />
{/* Add more UI components and styling as needed */}
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
padding: 20,
},
label: {
fontSize: 18,
},
input: {
borderWidth: 1,
borderColor: '#ccc',
padding: 10,
marginBottom: 20,
},
});
export default SignUpScreen;
In this code sample, we create a simple sign-up form with email and password fields. When the “Sign Up” button is pressed, the handleSignUp
function is called. In a real-world scenario, you would typically validate the user’s input and make an API call to register the user. After successful registration, you can navigate to another screen using React Navigation.
React Native, “search” code sample
Creating a mobile search feature in a React Native application typically involves implementing a search input field and filtering or searching through a list of items. Here’s a simple code sample for a mobile search component using React Native:
jsxCopy codeimport React, { useState } from 'react';
import { View, Text, TextInput, FlatList, StyleSheet } from 'react-native';
const data = [
{ id: '1', name: 'Item 1' },
{ id: '2', name: 'Item 2' },
{ id: '3', name: 'Item 3' },
{ id: '4', name: 'Another Item' },
// Add more data items here
];
const SearchScreen = () => {
const [searchText, setSearchText] = useState('');
const filteredData = data.filter(item =>
item.name.toLowerCase().includes(searchText.toLowerCase())
);
return (
<View style={styles.container}>
<TextInput
style={styles.searchInput}
onChangeText={text => setSearchText(text)}
value={searchText}
placeholder="Search for items"
/>
<FlatList
data={filteredData}
keyExtractor={item => item.id}
renderItem={({ item }) => (
<Text style={styles.listItem}>{item.name}</Text>
)}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
padding: 20,
},
searchInput: {
borderWidth: 1,
borderColor: '#ccc',
padding: 10,
marginBottom: 20,
},
listItem: {
fontSize: 18,
marginBottom: 10,
},
});
export default SearchScreen;
In this code sample:
- We have a
data
array containing a list of items. - The
SearchScreen
component renders a search input field and a list of items. - The
filteredData
variable is used to filter the items based on thesearchText
state. It filters items whose names contain the search text (case-insensitive). - As you type in the search input, the list of items is dynamically filtered.