Contents
Swift development language features
Swift is a powerful and modern programming language developed by Apple for iOS, macOS, watchOS, tvOS, and more. It is designed to be easy to read, write, and maintain while also being safe, efficient, and versatile. Here are some of the key language features of Swift development language:
- Safety:
- Type Safety: Swift is a statically-typed language, which means variable types are checked at compile time, reducing runtime errors.
- Optionals: Optionals allow you to handle the absence of a value in a type-safe manner.
- Memory Safety: Swift uses automatic reference counting (ARC) to manage memory, preventing common memory-related bugs.
- Performance:
- Highly Optimized: Swift is designed for performance, with many language features aimed at minimizing runtime overhead.
- Low-Level Control: You can write high-performance code using low-level control constructs like pointers.
- Readability:
- Clean Syntax: Swift has a concise and expressive syntax, making it easier to read and write code.
- Inferred Types: Swift can often infer the data types of variables, reducing the need for explicit type declarations.
- Modern Features:
- Closures: Swift development language supports closures, which are similar to blocks in Objective-C. Closures allow you to define and pass around functions as variables.
- Generics: Swift development languageprovides a robust system for writing reusable, type-safe code through generics.
- Type Inference: The type of a variable or expression can often be inferred by the compiler, reducing the need for explicit type annotations.
- Pattern Matching: Swift includes powerful pattern matching for working with complex data structures.
- Interoperability:
- Objective-C Compatibility: Swift development language can work seamlessly with existing Objective-C code, and you can even use Objective-C libraries in Swift.
- C/C++ Compatibility: You can use Swift with C and C++ code by creating bridging headers.
- Concurrency:
- Async/Await: Swift 5.5 introduced async/await to make asynchronous programming more natural and less error-prone.
- Actors: Actors are used for safe concurrent access to mutable state.
- Multiple Paradigms:
- Swift development language supports both object-oriented and functional programming paradigms, making it versatile for different coding styles.
- Error Handling:
- Swift development language uses a robust error-handling model with the
try
,catch
, andthrow
keywords.
- Swift development language uses a robust error-handling model with the
- Automatic Reference Counting (ARC):
- ARC manages memory for you, automatically deallocating objects when they are no longer needed.
- String Interpolation:
- Swift development language allows you to easily interpolate variables and expressions into string literals.
- Playgrounds:
- Xcode provides an interactive playground environment where you can experiment with Swift code and see results in real-time.
- Package Management:
- Swift development language Package Manager is a built-in tool for managing dependencies and building Swift projects.
These features, among others, make Swift development language a popular choice for developing iOS and macOS applications, as well as for server-side development and other domains. It combines the best aspects of modern programming languages while addressing many of the shortcomings of Objective-C.
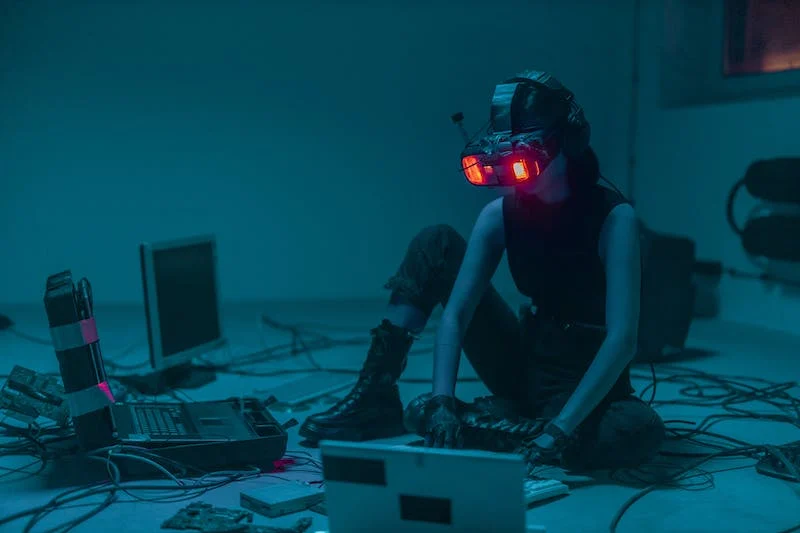
Swift development language mobile “sign-up” code sample
Creating a complete Swift mobile sign-up code sample is beyond the scope of a simple response, as it involves various components, such as UI design, form validation, network requests, and data storage. However, I can provide you with a simplified example of how you might implement a basic sign-up screen in Swift.
Below is a Swift code sample for a simple sign-up view controller. This example assumes that you have a UI built in a storyboard and connected to your view controller. In a real-world application, you’d also want to implement proper error handling, input validation, and a network request to create the user account.
swiftCopy codeimport UIKit
class SignupViewController: UIViewController {
// Connect these outlets to your UI elements in the storyboard
@IBOutlet weak var usernameTextField: UITextField!
@IBOutlet weak var passwordTextField: UITextField!
@IBOutlet weak var confirmPasswordTextField: UITextField!
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func signUpButtonTapped(_ sender: UIButton) {
// Perform sign-up action when the sign-up button is tapped
let username = usernameTextField.text ?? ""
let password = passwordTextField.text ?? ""
let confirmPassword = confirmPasswordTextField.text ?? ""
// Perform input validation (e.g., checking if passwords match)
if password.isEmpty || confirmPassword.isEmpty || password != confirmPassword {
// Handle validation error (display an alert, for example)
showValidationError(message: "Passwords do not match.")
return
}
// Assuming you have a function for making a network request to sign up the user
signUp(username: username, password: password)
}
func signUp(username: String, password: String) {
// Replace this with your actual API request code
// Make an API request to sign up the user
// You may use URLSession or a networking library like Alamofire
// Handle success and error responses
// For simplicity, this example just shows an alert
let alert = UIAlertController(title: "Sign-Up Success", message: "User successfully signed up!", preferredStyle: .alert)
alert.addAction(UIAlertAction(title: "OK", style: .default, handler: nil))
present(alert, animated: true, completion: nil)
}
func showValidationError(message: String) {
let alert = UIAlertController(title: "Validation Error", message: message, preferredStyle: .alert)
alert.addAction(UIAlertAction(title: "OK", style: .default, handler: nil)
present(alert, animated: true, completion: nil)
}
}
In a real app, you’d need to handle network requests more securely, possibly using HTTPS, and implement robust error handling, user feedback, and securely storing user credentials. You’d also typically involve a server-side component for user management.
Additionally, consider using a library like Alamofire
or URLSession
for handling network requests, and follow best practices for securing user data and managing user accounts.
Swift development language mobile “search” code
Creating a complete Swift code sample for a mobile search functionality depends on your specific use case, such as searching for data in a table view, filtering results, and more. Below is a simplified example of how you might implement a basic search feature in Swift within a UITableView
. This example assumes that you have a table view and a search bar set up in your view controller.
swiftCopy codeimport UIKit
class SearchViewController: UIViewController, UITableViewDelegate, UITableViewDataSource, UISearchBarDelegate {
@IBOutlet weak var searchBar: UISearchBar!
@IBOutlet weak var tableView: UITableView!
var data = ["Apple", "Banana", "Cherry", "Date", "Elderberry", "Fig", "Grape", "Honeydew", "Kiwi", "Lemon", "Mango", "Orange", "Peach", "Pear", "Quince", "Raspberry", "Strawberry", "Tomato", "Watermelon"]
var filteredData: [String] = []
override func viewDidLoad() {
super.viewDidLoad()
// Set the delegate for the search bar
searchBar.delegate = self
// Set the delegate and data source for the table view
tableView.delegate = self
tableView.dataSource = self
}
// MARK: - UITableViewDataSource methods
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return filteredData.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath)
cell.textLabel?.text = filteredData[indexPath.row]
return cell
}
// MARK: - UISearchBarDelegate method
func searchBar(_ searchBar: UISearchBar, textDidChange searchText: String) {
filteredData = data.filter { $0.lowercased().contains(searchText.lowercased) }
tableView.reloadData()
}
}