Contents
History of the Java development language
The history of the Java programming language is a fascinating journey that spans several decades and involves key developments, innovations, and contributions from various individuals and organizations.
- Origins (Early 1990s): The story of Java begins with James Gosling, Mike Sheridan, and Patrick Naughton, who were engineers at Sun Microsystems (now part of Oracle Corporation). In the early 1990s, they started a project called the “Green Project” to develop a new programming development language that could be used for embedded systems, consumer electronics, and other devices.
- Oak (1991): The original name of the development language was Oak, inspired by an oak tree that stood outside Gosling’s office window. Oak was designed for interactive television and set-top box applications. However, the name had to be changed due to trademark conflicts.
- Java (1995): In 1995, Sun Microsystems officially announced the Java programming development language. The name “Java” was chosen due to its association with the coffee that was popular at Sun’s headquarters. Java was positioned as a platform-independent, object-oriented programming language that could run on any device with a Java Virtual Machine (JVM).
- JVM (1995): Alongside the development language, Sun introduced the Java Virtual Machine (JVM), a critical component of the Java platform. The JVM allowed Java programs to be executed on different hardware and operating systems without modification.
- “Write Once, Run Anywhere” (1995): One of Java’s most significant features was its promise of “Write Once, Run Anywhere.” This meant that Java applications could be developed on one platform and run on any other platform with a compatible JVM. This portability was a game-changer for software development.
- Java 1.0 (1996): The first official version of Java, Java 1.0, was released in January 1996. It included the core features and libraries that laid the foundation for future versions of the development language.
- Java’s Popularity (Late 1990s – Early 2000s): Java quickly gained popularity in the late 1990s and early 2000s due to its portability, security features, and the growth of the internet. Java became a prominent development language for web development, and it played a significant role in the rise of enterprise-level applications.
- J2EE, J2SE, and J2ME (Late 1990s – Early 2000s): Sun introduced various editions of Java to cater to different application domains, including Java 2 Platform, Enterprise Edition (J2EE), Java 2 Platform, Standard Edition (J2SE), and Java 2 Platform, Micro Edition (J2ME). Each edition was tailored for specific use cases.
- Open Sourcing Java (2006): In an effort to foster community involvement and development, Sun Microsystems open-sourced Java under the GNU General Public License (GPL) with the release of Java SE 6 in 2006. This move led to the creation of the OpenJDK (Open Java Development Kit).
- Oracle’s Acquisition (2010): Oracle Corporation acquired Sun Microsystems in 2010, becoming the steward of the Java platform. This acquisition led to some legal battles, most notably with Google over the use of Java in Android.
- Recent Java Versions: Java has continued to evolve with regular releases, introducing new features and improvements. Some notable versions include Java 8 with lambda expressions, Java 9 with the module system, Java 11 as a long-term support (LTS) release, and subsequent LTS releases.
- Project Loom and Valhalla (Ongoing): As of my last knowledge update in September 2021, Java was actively working on projects like Project Loom (focused on improving concurrency with fibers) and Project Valhalla (aimed at enhancing the JVM’s support for value types). These projects were expected to shape the future of Java development.
Java remains a widely used and influential programming development language in various domains, including web development, mobile app development, server-side applications, and more. Its community and ecosystem continue to thrive, with contributions from developers and organizations worldwide.
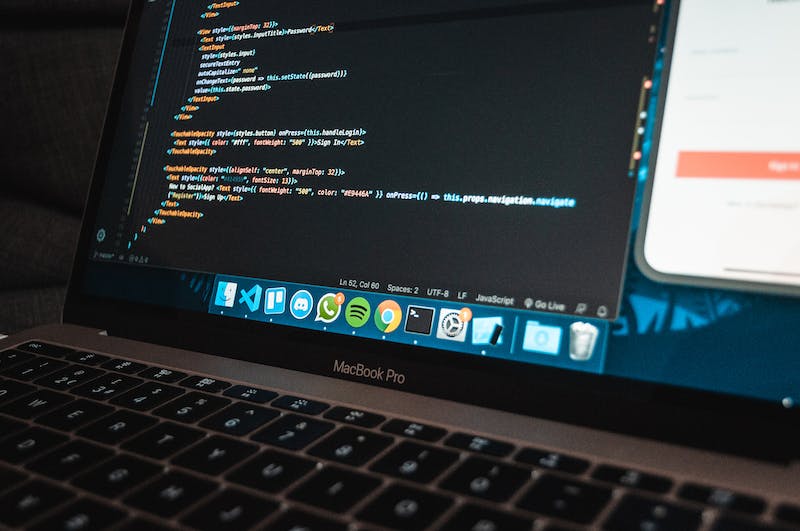
Features of Java development language
Java is a versatile and widely used programming development language known for its portability, platform independence, and robust features.
- Platform Independence: One of the most significant features of Java is its platform independence. Java code can be written once and run on any platform with a compatible Java Virtual Machine (JVM). This “Write Once, Run Anywhere” capability makes Java suitable for cross-platform development.
- Object-Oriented: Java is a purely object-oriented programming development language. It encourages the use of objects and classes, making it easy to organize and structure code.
- Strongly Typed: Java enforces strong data typing, which helps catch type-related errors at compile-time rather than at runtime. This enhances code reliability and robustness.
- Automatic Memory Management: Java includes an automatic garbage collector that manages memory allocation and deallocation. This simplifies memory management, reducing the risk of memory leaks and crashes due to memory-related issues.
- Robustness: Java’s design principles, including strong typing, exception handling, and memory management, contribute to the development language’s robustness. Java applications are less prone to crashes and errors.
- Security: Java has built-in security features, such as the sandboxing of applets in web browsers and the ability to define access controls and permissions. This makes Java suitable for building secure applications, especially in the context of web and enterprise software.
- Multi-Threading: Java provides extensive support for multi-threading, allowing developers to create concurrent and parallel applications easily. The
java.util.concurrent
package offers a range of tools for managing threads and synchronization. - Rich Standard Library: Java comes with a comprehensive standard library (Java Standard Library) that provides a wide range of classes and packages for various tasks, including data manipulation, networking, I/O, and GUI development.
- Portability: Java’s platform independence and bytecode compilation enable developers to write code on one platform and run it on multiple platforms without modification.
- Community and Ecosystem: Java has a large and active developer community, which has contributed to the creation of numerous libraries, frameworks, and tools. Popular Java frameworks include Spring, Hibernate, and Apache Struts.
- Backward Compatibility: Java maintains a strong commitment to backward compatibility, ensuring that code written in older versions of the development language remains functional in newer Java releases. This helps protect the investment in existing Java applications.
- High Performance: While Java is often associated with being an interpreted development language due to its bytecode execution, Just-In-Time (JIT) compilers can dynamically optimize code at runtime, making Java applications perform well.
- Scalability: Java is suitable for building both small-scale and large-scale applications. Its support for modularization and various frameworks allows developers to scale their projects effectively.
- Community-Driven: The Java community plays a significant role in the language’s development and evolution. Contributions to the OpenJDK project, discussions in various forums, and participation in Java User Groups (JUGs) help shape the language’s future.
These features have contributed to Java’s enduring popularity and its continued use in a wide range of application domains, from web and mobile development to enterprise software and embedded systems.
Coding examples for Java development language
1. Hello, World!
javaCopy codepublic class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
This is a simple Java program that prints “Hello, World!” to the console. It demonstrates the basic structure of a Java class and the main
method.
2. Variables and Data Types
javaCopy codepublic class VariablesExample {
public static void main(String[] args) {
// Declare and initialize variables
int age = 25;
double height = 5.9;
String name = "John";
// Display values
System.out.println("Name: " + name);
System.out.println("Age: " + age);
System.out.println("Height: " + height + " feet");
}
}
This example shows how to declare and use variables with different data types, including int
, double
, and String
.
3. Conditional Statements
javaCopy codepublic class ConditionalExample {
public static void main(String[] args) {
int number = 10;
if (number > 0) {
System.out.println("Number is positive.");
} else if (number < 0) {
System.out.println("Number is negative.");
} else {
System.out.println("Number is zero.");
}
}
}
Here, we use an if-else
statement to check whether a number is positive, negative, or zero based on its value.
4. Loops
javaCopy codepublic class LoopExample {
public static void main(String[] args) {
// Print numbers from 1 to 5 using a for loop
for (int i = 1; i <= 5; i++) {
System.out.print(i + " ");
}
System.out.println(); // Newline
// Print even numbers from 2 to 10 using a while loop
int num = 2;
while (num <= 10) {
System.out.print(num + " ");
num += 2;
}
}
}
This code demonstrates for
and while
loops to iterate and print numbers in a specified range.
5. Arrays
javaCopy codepublic class ArrayExample {
public static void main(String[] args) {
// Declare and initialize an array of integers
int[] numbers = {1, 2, 3, 4, 5};
// Access and print array elements
for (int i = 0; i < numbers.length; i++) {
System.out.print(numbers[i] + " ");
}
}
}