Contents
History of the Python development language
Python is a popular high-level programming language known for its simplicity and readability.
- Birth of Python (Late 1980s): Python development language was created by Guido van Rossum, a Dutch programmer, in the late 1980s. His motivation was to develop a language that would emphasize code readability and allow programmers to express their concepts in fewer lines of code than languages like C or C++. The first Python release, Python 0.9.0, was released in February 1991.
- Python 1.0 (January 1994): Python development language 1.0 was the first official version of Python and included many of the features that are still part of the language today, such as exception handling, functions, and modules.
- Python 2.0 (October 2000): Python development language 2.0 introduced list comprehensions and garbage collection. It was a significant release that further improved the language.
- Python 3.0 (December 2008): Python development language 3.0, also known as “Python 3000” or “Py3k,” marked a major milestone in Python’s development. It aimed to clean up and simplify the language by removing old and redundant features. This led to some backward incompatibilities with Python 2.x, which prompted a transition period.
- Python 2 vs. Python 3: The transition from Python development language 2 to Python 3 was a significant event in Python’s history. Python 2 continued to be maintained for a while to support legacy code, but its end-of-life (EOL) was officially declared for January 1, 2020. Python 3 gained widespread adoption and became the standard.
- Python Software Foundation (PSF): The Python development language Software Foundation, established in 2001, is a non-profit organization that manages Python’s development and promotes its use. It plays a crucial role in funding and supporting Python’s growth.
- Python Enhancement Proposals (PEP): PEPs are documents that describe proposed new features, APIs, and language modifications. The Python community uses PEPs to discuss and reach a consensus on the language’s development direction.
- Python 3.x Releases: After Python 3.0, several 3.x versions have been released with incremental improvements and new features. Python’s development cycle includes regular releases to introduce enhancements, bug fixes, and optimizations. Some notable versions include Python 3.5, Python 3.6, Python 3.7, Python 3.8, Python 3.9, and Python 3.10 (as of my last knowledge update in September 2021).
- Python’s Popularity and Ecosystem: Python’s simplicity, readability, and extensive libraries have contributed to its widespread popularity. It is widely used for web development (Django, Flask), data analysis (NumPy, pandas), machine learning and artificial intelligence (TensorFlow, PyTorch), and scientific computing, among other domains.
- Future Development: Python’s development continues with a focus on improving performance, language features, and maintaining backward compatibility. The Python community actively contributes to its growth, and PEPs are used to propose and discuss changes.
Python’s history is a testament to its evolution as a versatile and powerful programming language, with a strong and vibrant community of developers and users worldwide. The language continues to adapt and thrive in various application domains, making it an essential tool in modern software development.
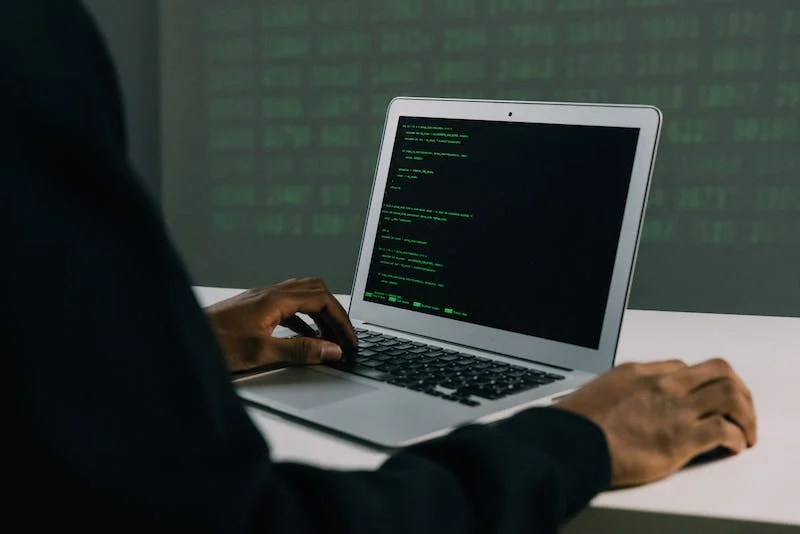
Features of the Python
Python is a versatile and powerful programming language known for its simplicity, readability, and a wide range of features. Here are some of the key features of the Python programming language:
- Easy to Read and Write: Python development language’s syntax is clear and easy to read, which makes it an excellent choice for beginners and experienced developers alike. It uses indentation to define code blocks, eliminating the need for curly braces or other delimiters.
- High-Level Language: Python development language is a high-level programming language, which means it abstracts complex low-level details like memory management and allows programmers to focus on solving problems more efficiently.
- Interpreted Language: Python development language is an interpreted language, which means you can write and execute code without the need for a separate compilation step. This makes development faster and more interactive.
- Cross-Platform: Python development language is available on various platforms, including Windows, macOS, and Linux, making it easy to write code that works across different operating systems.
- Dynamic Typing: Python development language uses dynamic typing, which means you don’t need to declare variable types explicitly. Variables can change types during runtime, offering flexibility and reducing the likelihood of type-related errors.
- Strongly Typed: Despite dynamic typing, Python is a strongly typed language, which means it enforces type constraints at runtime, helping to catch errors early.
- Extensive Standard Library: Python development language comes with a comprehensive standard library that includes modules for tasks like file I/O, regular expressions, networking, and more. This library reduces the need for third-party dependencies in many cases.
- Third-Party Libraries and Frameworks: Python has a vast ecosystem of third-party libraries and frameworks for various domains, such as web development (Django, Flask), scientific computing (NumPy, SciPy), data analysis (pandas), machine learning (TensorFlow, PyTorch), and more. These libraries extend Python’s capabilities significantly.
- Object-Oriented: Python supports object-oriented programming (OOP) principles, allowing you to define classes, create objects, and use inheritance and encapsulation to structure your code.
- Functional Programming: Python also supports functional programming concepts, such as first-class functions, lambda expressions, and higher-order functions.
- Easy Integration: Python can easily integrate with other languages like C, C++, and Java, which allows you to leverage existing codebases and libraries written in those languages.
- Community and Documentation: Python has a vibrant and supportive community of developers. There is extensive documentation, tutorials, and forums available, making it easy to learn and find help when needed.
- Open Source: Python is open source, meaning it is freely available, and developers can contribute to its development and improvement.
- Scalability: While Python may not be as performant as some lower-level languages, it is still capable of handling large-scale applications and systems when used in combination with appropriate tools and optimization techniques.
- Versatile Use Cases: Python is suitable for a wide range of applications, including web development, data analysis, scientific computing, automation, artificial intelligence, machine learning, scripting, and more.
These features make Python a popular choice for developers across various domains and contribute to its continued growth and adoption in the software development industry.
Coding examples for the Python development language
1. Hello, World!
pythonCopy codeprint("Hello, World!")
This classic “Hello, World!” program simply prints the message to the console.
2. Variables and Arithmetic Operations:
pythonCopy code# Variables
a = 5
b = 3
# Arithmetic operations
sum_result = a + b
difference_result = a - b
product_result = a * b
division_result = a / b
print("Sum:", sum_result)
print("Difference:", difference_result)
print("Product:", product_result)
print("Division:", division_result)
This code demonstrates the use of variables and basic arithmetic operations.
3. Conditional Statements:
pythonCopy code# Conditional statements
x = 10
if x > 5:
print("x is greater than 5")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than 5")
This example shows how to use if
, elif
, and else
for conditional branching.
4. Loops:
a. For Loop:
pythonCopy code# For loop
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
This code uses a for
loop to iterate over a list of fruits.
b. While Loop:
pythonCopy code# While loop
count = 0
while count < 5:
print("Count:", count)
count += 1
This code uses a while
loop to print numbers from 0 to 4.
5. Functions:
pythonCopy code# Function definition
def greet(name):
return "Hello, " + name + "!"
# Function call
message = greet("Alice")
print(message)
This code defines a simple function greet
that takes a name as an argument and returns a greeting message.
6. Lists and List Operations:
pythonCopy code# Lists and list operations
numbers = [1, 2, 3, 4, 5]
# Accessing elements
print("First element:", numbers[0])
print("Last element:", numbers[-1])
# Slicing
sliced_list = numbers[1:4]
print("Sliced list:", sliced_list)
# Adding an element
numbers.append(6)
print("Updated list:", numbers)
# Length of the list
list_length = len(numbers)
print("Length of the list:", list_length)