Contents
History of the C language
The C language has a rich history that dates back to the early 1970s. It was created by Dennis Ritchie at Bell Labs (formerly AT&T Bell Laboratories) in the United States.
- Origins (Early 1970s):
- C language’s development began in 1972 when Dennis Ritchie started working on a new programming language at Bell Labs. He was inspired by an earlier language called B, which had been developed by Ken Thompson.
- Development and Refinement (Early to Mid-1970s):
- Ritchie and his colleagues at Bell Labs continued to develop and refine C throughout the early to mid-1970s.
- The language was initially used for implementing the Unix operating system, which was also being developed at Bell Labs. C was well-suited for systems programming tasks.
- Release of the C Language (1973):
- The first version of the C programming language, known as “C73,” was released in 1973. This version was relatively simple and limited in its features.
- Standardization (Late 1970s – Early 1980s):
- In the late 1970s and early 1980s, C language underwent standardization efforts. The most notable result was the publication of “The C Programming Language,” commonly referred to as K&R C (named after the authors, Brian Kernighan and Dennis Ritchie), in 1978. This book became the de facto standard for the C language.
- ANSI C (1983):
- The American National Standards Institute (ANSI) began formal standardization of the C language in the early 1980s. This effort led to the publication of the ANSI C standard in 1983. ANSI C language introduced important features, including function prototypes, the
<stdio.h>
library, and more.
- The American National Standards Institute (ANSI) began formal standardization of the C language in the early 1980s. This effort led to the publication of the ANSI C standard in 1983. ANSI C language introduced important features, including function prototypes, the
- C89 (1989):
- The ANSI C language standard was updated in 1989 and became known as “C89” or “ANSI C89.” This version further refined and standardized the language.
- C99 (1999):
- In 1999, the C language standard was updated again to include new features and improvements. This version is commonly referred to as “C99.”
- C11 (2011):
- The next major revision of the C language standard, known as “C11,” was released in 2011. It introduced additional features, such as multithreading support and improved support for complex numbers.
- C18 (2018):
- The most recent version of the C language standard, “C18,” was published in 2018. It includes minor updates and clarifications but does not introduce significant changes to the language.
Throughout its history, the C programming language has played a crucial role in the development of computer systems, operating systems, application software, and more. Its simplicity, efficiency, and portability have contributed to its enduring popularity, and C continues to be a widely used and influential language in the world of programming. Many modern programming languages, including C++, C#, and Objective-C, have their roots in C.
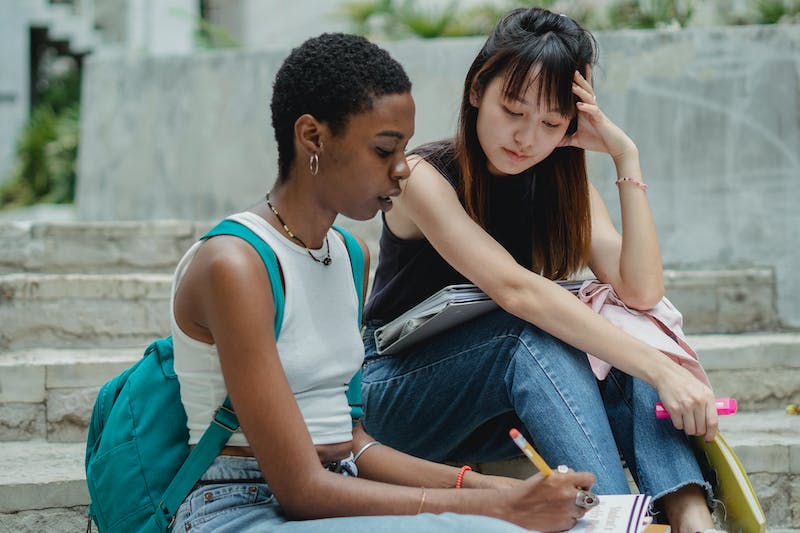
Features of the C development language
The C language is known for its simplicity and power. It provides a rich set of features that make it suitable for a wide range of applications, from systems programming to application development.
- Procedural Programming: C language is primarily a procedural programming language. It allows you to break down a program into functions or procedures, making it easier to manage and maintain code.
- Structured Language: C language supports structured programming with constructs like loops (for, while, do-while), conditionals (if-else), and functions, which promote code organization and readability.
- Low-Level Access: C provides low-level access to memory through pointers. This allows for efficient manipulation of data structures and direct interaction with hardware when needed.
- Efficiency: C is known for its efficiency and performance. It provides fine-grained control over memory allocation and allows for optimized code generation, making it suitable for systems programming and embedded systems.
- Portability: C programs are highly portable, meaning they can be compiled and run on different platforms with minimal changes. This portability is facilitated by the use of standard libraries and a small set of keywords.
- Rich Standard Library: C comes with a standard library (e.g.,
<stdio.h>
,<stdlib.h>
) that provides functions for input/output, memory allocation, string manipulation, and more. This library simplifies common programming tasks. - Static Typing: C is statically typed, which means variable types are determined at compile-time. This helps catch type-related errors before the program runs.
- Pointers: C supports pointers, which are variables that store memory addresses. Pointers are a powerful feature for working with arrays, structures, and dynamic memory allocation.
- User-Defined Data Types: C allows you to define custom data types using structures and unions. This enables the creation of complex data structures tailored to specific needs.
- Bit Manipulation: C provides bitwise operators and bit fields, making it suitable for tasks that involve low-level bit manipulation.
- Modularity: C supports modular programming through the use of header files and separate compilation. This encourages code reuse and maintainability.
- Recursion: C supports recursive functions, which allow a function to call itself. This is useful for solving problems that have a recursive nature, such as tree traversal.
- Library Support: C has a vast community of libraries and frameworks for various purposes, including graphics (e.g., OpenGL), networking (e.g., sockets), and more.
- Standardization: C has a well-defined standard, maintained by organizations like ANSI and ISO, which ensures language consistency and compatibility across different compilers.
- Flexibility: C provides flexibility to perform low-level operations when necessary, making it suitable for tasks like writing device drivers and operating systems.
- Community and Resources: C has a large and active community, which means there are plenty of resources, books, tutorials, and forums available for learning and getting help with C programming.
Despite its age, C remains a widely used and influential programming language, and its core features have influenced the design of many other programming languages. While it may not have the high-level abstractions of more modern languages, its simplicity and control make it a valuable tool for certain types of software development.
Coding examples in C development language
Certainly! Here are some simple coding examples in the C programming language to illustrate various aspects of C programming:
- Hello World Program:cCopy code
#include <stdio.h> int main() { printf("Hello, World!\n"); return 0; }
This is the classic “Hello, World!” program in C. It demonstrates basic input/output using theprintf
function. - Variables and Data Types:cCopy code
#include <stdio.h> int main() { int age = 25; float height = 175.5; char grade = 'A'; printf("Age: %d\n", age); printf("Height: %.1f\n", height); printf("Grade: %c\n", grade); return 0; }
This program shows how to declare and use variables of different data types. - User Input:cCopy code
#include <stdio.h> int main() { char name[50]; printf("Enter your name: "); scanf("%s", name); printf("Hello, %s!\n", name); return 0; }
It takes user input using thescanf
function. - Conditional Statements (if-else):cCopy code
#include <stdio.h> int main() { int num; printf("Enter a number: "); scanf("%d", &num); if (num > 0) { printf("The number is positive.\n"); } else if (num < 0) { printf("The number is negative.\n"); } else { printf("The number is zero.\n"); } return 0; }
Demonstrates conditional statements in C. - Loops (for and while):cCopy code
#include <stdio.h> int main() { // Using a for loop to print numbers from 1 to 5 for (int i = 1; i <= 5; i++) { printf("%d ", i); } printf("\n"); // Using a while loop to calculate the sum of numbers from 1 to 10 int sum = 0; int j = 1; while (j <= 10) { sum += j; j++; } printf("Sum of numbers from 1 to 10: %d\n", sum); return 0; }
Illustrates how to usefor
andwhile
loops. - Arrays:cCopy code
#include <stdio.h> int main() { int numbers[] = {1, 2, 3, 4, 5}; int sum = 0; for (int i = 0; i < 5; i++) { sum += numbers[i]; } printf("Sum of numbers in the array: %d\n", sum); return 0; }
Shows how to work with arrays.
These examples cover some fundamental aspects of C programming. You can build upon them to explore more advanced concepts like functions, pointers, structures, and file I/O as you become more proficient in C development.