Contents
Unsupervised Machine Learning programs
Unsupervised learning programs have several distinctive characteristics:
- No Labeled Output: In unsupervised learning, the algorithm does not receive labeled output or target values to guide its learning. Instead, it explores the inherent structure or patterns in the data on its own.
- Exploratory in Nature: Unsupervised learning is often used for exploratory data analysis. It helps discover hidden patterns, relationships, or clusters within the data that may not be apparent through manual inspection.
- Types of Unsupervised Learning:
- Clustering: Unsupervised learning can be used for clustering, where similar data points are grouped together into clusters. K-Means, Hierarchical Clustering, and DBSCAN are examples of clustering algorithms.
- Dimensionality Reduction: It can also be used for dimensionality reduction, where the goal is to reduce the number of features (variables) while preserving important information. Principal Component Analysis (PCA) and t-SNE are common techniques for dimensionality reduction.
- Anomaly Detection: Unsupervised learning can be used for anomaly or outlier detection, where the algorithm identifies data points that are significantly different from the majority of the data.
- Density Estimation: It can be used to estimate the probability density function of the data, which is useful in tasks like density-based clustering.
- No Explicit Feedback: Since there are no target labels, there’s no explicit feedback loop for the algorithm to minimize errors as in supervised learning. Instead, unsupervised learning often relies on heuristics and evaluation metrics specific to the task.
- Applications: Unsupervised learning has applications in various domains, including natural language processing, image and speech analysis, customer segmentation, recommendation systems, and anomaly detection.
- Evaluation: The evaluation of unsupervised learning models can be challenging because there are no clear targets for comparison. Evaluation often involves measures like silhouette score (for clustering), explained variance (for dimensionality reduction), or domain-specific metrics.
- Preprocessing and Feature Engineering: Data preprocessing, such as scaling, normalization, and handling missing values, is crucial in unsupervised learning. Feature engineering is also relevant, especially when performing dimensionality reduction.
- Model Selection: The choice of the appropriate unsupervised learning algorithm depends on the specific problem and the characteristics of the data. Different algorithms may be more suitable for clustering, dimensionality reduction, or other tasks.
- Interpretability: Interpreting the results of unsupervised learning can be challenging, especially in high-dimensional spaces. It often requires domain knowledge and visualization techniques to make sense of the discovered patterns.
- Unbiased Exploration: Unsupervised learning can provide an unbiased exploration of data, uncovering insights that might be missed through human intuition or bias.
In summary, unsupervised learning is a valuable branch of machine learning that focuses on discovering patterns and structures in data without the need for labeled output. It has a wide range of applications and is particularly useful for data exploration and preprocessing, as well as for solving specific tasks like clustering and dimensionality reduction.
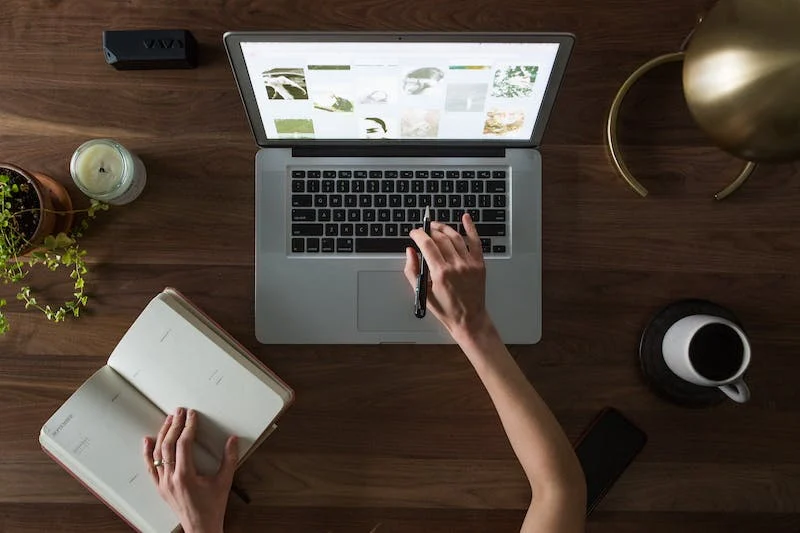
Machine Learning, Unsupervised learning coding
Here are some coding examples of unsupervised learning using Python and scikit-learn for common unsupervised learning tasks:
Example 1: K-Means Clustering
In this example, we’ll use the K-Means clustering algorithm to cluster data into groups based on their similarity. We’ll use the Iris dataset for this demonstration.
pythonCopy codefrom sklearn.datasets import load_iris
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
# Load the Iris dataset
data = load_iris()
X = data.data
# Instantiate a K-Means model with 3 clusters
kmeans = KMeans(n_clusters=3, random_state=42)
# Fit the model to the data
kmeans.fit(X)
# Get cluster labels
cluster_labels = kmeans.labels_
# Visualize the clusters (for 2D data, e.g., the first two features)
plt.scatter(X[:, 0], X[:, 1], c=cluster_labels, cmap='viridis')
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.show()
Example 2: Principal Component Analysis (PCA)
PCA is used for dimensionality reduction. In this example, we’ll reduce the dimensionality of the Iris dataset and visualize it in 2D.
pythonCopy codefrom sklearn.datasets import load_iris
from sklearn.decomposition import PCA
import matplotlib.pyplot as plt
# Load the Iris dataset
data = load_iris()
X = data.data
# Apply PCA to reduce to 2 dimensions
pca = PCA(n_components=2)
X_2d = pca.fit_transform(X)
# Visualize the reduced data
plt.scatter(X_2d[:, 0], X_2d[:, 1], c=data.target, cmap='viridis')
plt.xlabel('Principal Component 1')
plt.ylabel('Principal Component 2')
plt.show()
Example 3: Gaussian Mixture Model (GMM)
GMM is a probabilistic model that can be used for clustering. In this example, we’ll apply GMM to cluster data and visualize the results.
pythonCopy codeimport numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import make_blobs
from sklearn.mixture import GaussianMixture
# Generate synthetic data
X, _ = make_blobs(n_samples=300, centers=4, random_state=42)
# Fit a Gaussian Mixture Model with 4 components
gmm = GaussianMixture(n_components=4, random_state=42)
gmm.fit(X)
# Predict the cluster labels
cluster_labels = gmm.predict(X)
# Visualize the clusters
plt.scatter(X[:, 0], X[:, 1], c=cluster_labels, cmap='viridis')
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.show()
These examples demonstrate the application of unsupervised learning techniques, including clustering (K-Means, GMM) and dimensionality reduction (PCA), using scikit-learn in Python.