Contents
Supervised Learning Program
Supervised learning is a type of machine learning where an algorithm learns from labeled training data to make predictions or decisions without being explicitly programmed. In supervised learning, the training dataset consists of input-output pairs, where the input data is associated with the corresponding target labels or outputs.
- We import necessary libraries, including scikit-learn, which is a popular Python library for machine learning.
- We load a sample dataset (the Iris dataset) using scikit-learn. This dataset contains features (sepal length, sepal width, petal length, and petal width) of iris flowers and their corresponding target labels (species: setosa, versicolor, or virginica).
- We split the dataset into training and testing sets using the
train_test_split
function. This helps us evaluate the model’s performance on unseen data. - We create a K-Nearest Neighbors (KNN) classifier using
KNeighborsClassifier
from scikit-learn. - We train the KNN classifier on the training data using the
fit
method. - We use the trained model to make predictions on the test data.
- We calculate the accuracy of the classifier by comparing the predicted labels (
y_pred
) to the actual labels (y_test
) using theaccuracy_score
function.
This is a simple example of a supervised learning program using scikit-learn. In practice, you can apply supervised learning to various types of data and problems by choosing different algorithms and preprocessing techniques based on the specific task you want to solve.
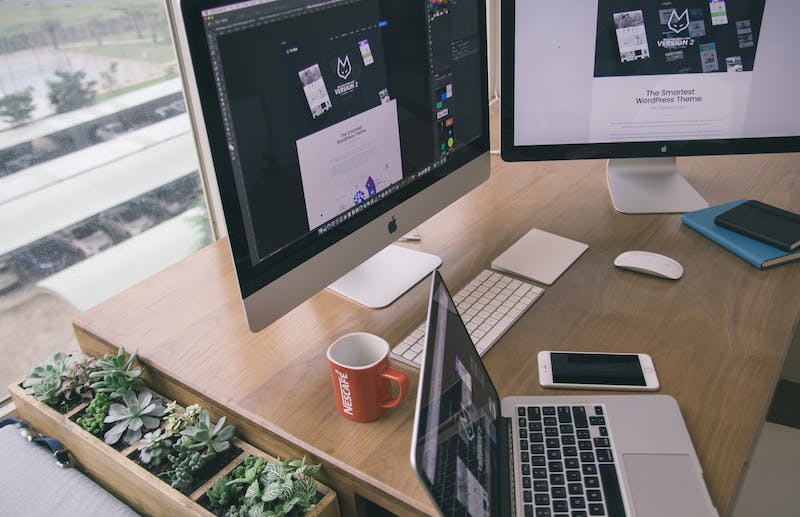
Machine Learning, Supervised Learning Coding Examples
Here are some examples of supervised learning coding examples using Python and scikit-learn, a popular machine learning library. These examples cover different types of supervised learning tasks, including classification and regression.
Example 1: Binary Classification with Logistic Regression
In this example, we’ll use logistic regression for binary classification on the famous Iris dataset:
pythonCopy codefrom sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Load the Iris dataset
data = load_iris()
X = data.data
y = (data.target == 0).astype(int) # Setosa vs. non-Setosa
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train a logistic regression model
model = LogisticRegression()
model.fit(X_train, y_train)
# Make predictions on the test set
y_pred = model.predict(X_test)
# Calculate accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy:.2f}')
Example 2: Multiclass Classification with Random Forest
In this example, we’ll perform multiclass classification using a Random Forest classifier on the Iris dataset:
pythonCopy codefrom sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load the Iris dataset
data = load_iris()
X = data.data
y = data.target
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train a Random Forest classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions on the test set
y_pred = model.predict(X_test)
# Calculate accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy:.2f}')
Example 3: Regression with Linear Regression
In this example, we’ll perform regression using a Linear Regression model on a synthetic dataset:
pythonCopy codeimport numpy as np
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Generate synthetic data
np.random.seed(0)
X = 2 * np.random.rand(100, 1)
y = 4 + 3 * X + np.random.randn(100, 1)
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train a Linear Regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions on the test set
y_pred = model.predict(X_test)
# Calculate mean squared error (a regression metric)
mse = mean_squared_error(y_test, y_pred)
print(f'Mean Squared Error: {mse:.2f}')
These examples illustrate how to perform various supervised learning tasks using scikit-learn. Depending on your specific problem, you can choose different algorithms and libraries, but scikit-learn is an excellent starting point for many supervised learning tasks in Python.