Contents
RubyMotion development language features
RubyMotion is a development platform that allows you to write native mobile applications for iOS, macOS, Android, and watchOS using the Ruby programming language. It provides a set of tools and libraries that enable you to build apps that perform and look like native applications on each platform. Here are some of the key language features and concepts in RubyMotion development:
- Ruby Programming Language: RubyMotion uses the Ruby programming language, which is known for its simplicity and readability. This means you can leverage Ruby’s syntax and features while developing mobile apps.
- Native UI: RubyMotion allows you to create native user interfaces for each platform. You can use platform-specific components and design principles to ensure your app looks and feels like a native application.
- Language Bridge: RubyMotion provides a bridge between Ruby and the platform-specific languages (Objective-C for iOS/macOS and Java for Android) so that you can access platform APIs and libraries seamlessly.
- iOS, macOS, and Android Support: RubyMotion supports development for multiple platforms, including iOS, macOS, and Android. You can write code once and target multiple platforms.
- Integrated Development Environment (IDE): RubyMotion offers an integrated development environment called RubyMine, which is a popular Ruby and Rails IDE. It provides features like code completion, debugging, and project management to streamline the development process.
- Libraries and Gems: RubyMotion allows you to use Ruby gems (libraries) as well as platform-specific libraries and frameworks. This makes it easy to extend the functionality of your mobile applications.
- Building and Packaging: You can use RubyMotion to build and package your applications for distribution on the respective app stores, such as the Apple App Store and Google Play Store.
- Testing and Debugging: RubyMotion provides tools for testing and debugging your applications, including support for unit tests and interactive debugging.
- Community and Documentation: RubyMotion has an active community of developers, and there is documentation and tutorials available to help you get started and troubleshoot issues.
- Cross-Platform Code Sharing: While RubyMotion allows you to target multiple platforms with a single codebase, you may still need to write platform-specific code for some components or interactions. However, you can often share a significant portion of your code across platforms.
Overall, RubyMotion simplifies the process of creating native mobile applications by leveraging the power and elegance of the Ruby programming language while allowing you to access and integrate with platform-specific features and APIs.
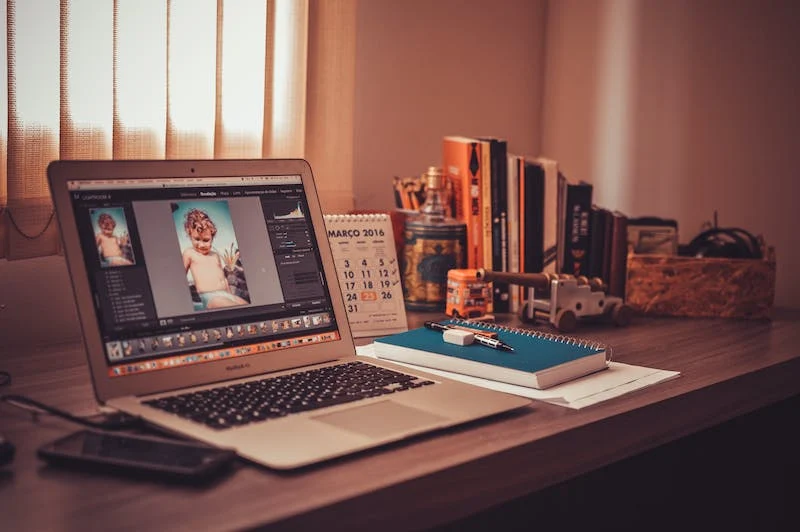
RubyMotion, mobile sign-up code sample
Creating a complete mobile sign-up application in RubyMotion is a substantial project, but I can provide you with a basic code sample to give you an idea of how to start building a sign-up form in RubyMotion for iOS. In this example, I’ll use the RubyMotion platform and the built-in UI components to create a simple sign-up screen. Please note that this is a minimal example, and in a real-world application, you would need to handle user input validation, data storage, and more.
rubyCopy codeclass SignupScreen < UIViewController
def viewDidLoad
super
self.title = "Sign Up"
view.backgroundColor = UIColor.whiteColor
@username_field = UITextField.new
@username_field.placeholder = "Username"
@username_field.borderStyle = UITextBorderStyleRoundedRect
@username_field.frame = [[20, 100], [280, 40]]
view.addSubview(@username_field)
@email_field = UITextField.new
@email_field.placeholder = "Email"
@email_field.borderStyle = UITextBorderStyleRoundedRect
@email_field.frame = [[20, 150], [280, 40]]
view.addSubview(@email_field)
@password_field = UITextField.new
@password_field.placeholder = "Password"
@password_field.secureTextEntry = true
@password_field.borderStyle = UITextBorderStyleRoundedRect
@password_field.frame = [[20, 200], [280, 40]]
view.addSubview(@password_field)
@signup_button = UIButton.buttonWithType(UIButtonTypeSystem)
@signup_button.setTitle("Sign Up", forState: UIControlStateNormal)
@signup_button.frame = [[20, 250], [280, 40]]
@signup_button.addTarget(self, action: 'signup', forControlEvents: UIControlEventTouchUpInside)
view.addSubview(@signup_button)
end
def signup
# Implement your sign-up logic here
# This is where you would send the data to your server or save it locally
# You would typically perform data validation and error handling as well
end
end
In this code:
- We create a
SignupScreen
class, which is aUIViewController
responsible for displaying the sign-up form. - In the
viewDidLoad
method, we set up the UI components for username, email, password fields, and a sign-up button. - The
signup
method is called when the sign-up button is tapped. You would implement your sign-up logic within this method, such as sending data to a server or saving it locally.
RubyMotion, mobile search code sample
Creating a complete mobile search application in RubyMotion is a more involved project, but I can provide you with a basic code sample to help you get started with building a search feature in a mobile app. In this example, I’ll demonstrate how to create a simple search interface using the RubyMotion platform for iOS. Please keep in mind that this is a basic illustration, and a real-world search functionality might require more features, data handling, and UI components.
Assuming you have a RubyMotion project set up, you can create a simple search interface with the following code:
rubyCopy codeclass SearchScreen < UIViewController
def viewDidLoad
super
self.title = "Search"
view.backgroundColor = UIColor.whiteColor
@search_bar = UISearchBar.alloc.initWithFrame(CGRectMake(0, 0, self.view.frame.size.width, 44))
@search_bar.delegate = self
view.addSubview(@search_bar)
@table_view = UITableView.alloc.initWithFrame([[0, 44], [self.view.frame.size.width, self.view.frame.size.height - 44]])
@table_view.dataSource = self
@table_view.delegate = self
view.addSubview(@table_view)
@data = ["Apple", "Banana", "Cherry", "Date", "Fig", "Grape", "Lemon", "Mango", "Orange", "Pineapple"]
@filtered_data = @data
end
def searchBar(searchBar, textDidChange: searchText)
if searchText.empty?
@filtered_data = @data
else
@filtered_data = @data.select { |item| item.downcase.include?(searchText.downcase) }
end
@table_view.reloadData
end
# UITableViewDataSource methods
def tableView(tableView, numberOfRowsInSection: section)
@filtered_data.length
end
def tableView(tableView, cellForRowAtIndexPath: indexPath)
cell = tableView.dequeueReusableCellWithIdentifier("Cell") || begin
UITableViewCell.alloc.initWithStyle(UITableViewCellStyleDefault, reuseIdentifier: "Cell")
end
cell.textLabel.text = @filtered_data[indexPath.row]
cell
end
# UITableViewDelegate methods
def tableView(tableView, didSelectRowAtIndexPath: indexPath)
selected_item = @filtered_data[indexPath.row]
puts "Selected: #{selected_item}"
end
end
In this code:
- We create a
SearchScreen
class, which is aUIViewController
responsible for displaying the search interface. - In the
viewDidLoad
method, we set up the UI components, including aUISearchBar
and aUITableView
. We also initialize an array of sample data. - The
searchBar
method is called when the user types in the search bar. It filters the data based on the search text and updates the table view accordingly. - We implement the
UITableViewDataSource
andUITableViewDelegate
methods to populate the table view with the filtered data and handle row selection.