Contents
Differences between C development language and C++ development language
C and C++ are both popular programming languages, and while they share many similarities, they also have several key differences:
- Paradigm:
- C development language is a procedural programming language, which means it focuses on procedures or functions to execute tasks in a linear fashion.
- C++development language is a multi-paradigm language that supports both procedural and object-oriented programming (OOP) paradigms. It introduces the concept of classes and objects, enabling developers to create reusable and organized code through encapsulation, inheritance, and polymorphism.
- Objects and Classes:
- C development language does not have built-in support for classes or objects. It primarily deals with functions, data types, and structures.
- C++ development language introduces the concept of classes and objects, allowing developers to model real-world entities with data and functions that operate on that data. This OOP feature is a significant distinction from C.
- Standard Libraries:
- C development language has a minimalistic standard library compared to C++. It provides basic functionality for file I/O, memory manipulation, and other low-level operations.
- C++ development language extends the C standard library with the Standard Template Library (STL), which provides a rich collection of data structures (like vectors, lists, and maps) and algorithms (such as sorting and searching) that can be used with minimal effort.
- Memory Management:
- In C development language, memory management is primarily manual, and developers must explicitly allocate and deallocate memory using functions like
malloc
andfree
. - C++ development language includes features like constructors and destructors, which can be used to manage resources (including memory) automatically through mechanisms like RAII (Resource Acquisition Is Initialization). C++ also has the
new
anddelete
operators for dynamic memory allocation.
- In C development language, memory management is primarily manual, and developers must explicitly allocate and deallocate memory using functions like
- Function Overloading:
- C development language does not support function overloading, which means you cannot have multiple functions with the same name but different parameter lists.
- C++ development language supports function overloading, allowing you to define multiple functions with the same name as long as their parameter lists differ.
- Operator Overloading:
- C does not support operator overloading, so you cannot redefine the behavior of operators for custom data types.
- C++ allows you to overload operators for user-defined types, which can make code more intuitive and readable.
- Exception Handling:
- C does not have built-in exception handling mechanisms.
- C++ provides exception handling using
try
,catch
, andthrow
constructs, making it easier to handle and propagate errors.
- Compatibility:
- C++ is largely compatible with C. You can often compile C code with a C++ compiler, and C functions can be called from C++ code. However, C++ introduces features and syntax that are not present in C, so pure C code may not be directly compatible with C++.
Overall, the choice between C and C++ depends on your specific project requirements and coding preferences. C is often chosen for systems programming, embedded systems, and low-level programming tasks, while C++ is favored for larger, more complex applications and projects that benefit from OOP features and the STL.
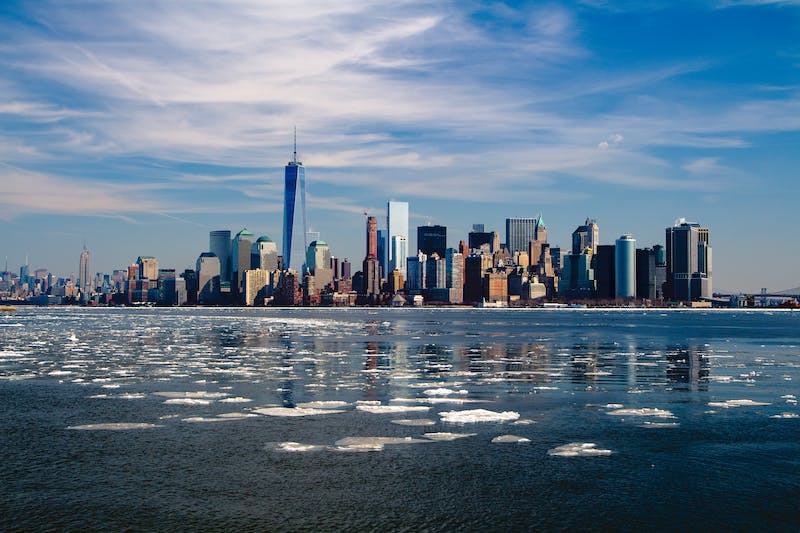
Coding examples for the C++
Certainly! Here are some simple C++ code examples to demonstrate various aspects of the language, including basic syntax, variables, input/output, loops, conditionals, functions, and classes:
- Hello World:cppCopy code
#include <iostream> using namespace std; int main() { cout << "Hello, World!" << endl; return 0; }
- Variables and Input/Output:cppCopy code
#include <iostream> using namespace std; int main() { int age; cout << "Enter your age: "; cin >> age; cout << "You are " << age << " years old." << endl; return 0; }
- Loops (for and while):cppCopy code
#include <iostream> using namespace std; int main() { // For loop for (int i = 1; i <= 5; i++) { cout << i << " "; } cout << endl; // While loop int j = 1; while (j <= 5) { cout << j << " "; j++; } cout << endl; return 0; }
- Conditional Statements (if-else):cppCopy code
#include <iostream> using namespace std; int main() { int number; cout << "Enter a number: "; cin >> number; if (number % 2 == 0) { cout << "Even" << endl; } else { cout << "Odd" << endl; } return 0; }
- Functions:cppCopy code
#include <iostream> using namespace std; // Function declaration int add(int a, int b); int main() { int result = add(3, 4); cout << "Sum: " << result << endl; return 0; } // Function definition int add(int a, int b) { return a + b; }
- Classes and Objects:cppCopy code
#include <iostream> using namespace std; class Rectangle { public: int length; int width; int area() { return length * width; } }; int main() { Rectangle rect; rect.length = 5; rect.width = 3; cout << "Area: " << rect.area() << endl; return 0; }
These are basic examples to get you started with C++. Depending on your goals and the complexity of your project, you can explore more advanced topics like inheritance, polymorphism, templates, and the Standard Template Library (STL) to build more sophisticated C++ applications.